-
public class BaseCustomControl : TextBox
{
public string InvalidRequiredMessage
{
get
{
string s = "Required";
object obj = ViewState["InvalidRequiredMessage"];
if (obj != null)
{
s = (string)obj;
}
return s;
}
set
{
ViewState["InvalidRequiredMessage"] = value;
}
}
public bool EnableClientScript
{
get
{
bool b = true;
object obj = ViewState["EnableClientScript"];
if (obj != null)
{
b = (bool)obj;
}
return b;
}
set
{
ViewState["EnableClientScript"] = value;
}
}
public bool IsRequired
{
get
{
bool b = true;
object obj = ViewState["IsRequired"];
if (obj != null)
{
b = (bool)obj;
}
return b;
}
set
{
ViewState["IsRequired"] = value;
}
}
public string CssClassTxt
{
get
{
string s = "txtCls";
object obj = ViewState["CssClassTxt"];
if (obj != null)
{
s = (string)obj;
}
return s;
}
set
{
ViewState["CssClassTxt"] = value;
this.CssClass = value;
}
}
public string CssClassErr
{
get
{
string s = "errCls";
object obj = ViewState["CssClassErr"];
if (obj != null)
{
s = (string)obj;
}
return s;
}
set
{
ViewState["CssClassErr"] = value;
}
}
protected override void OnInit(EventArgs e)
{
//check Required
if (IsRequired)
{
req = new RequiredFieldValidator();
req.ControlToValidate = this.ID;
req.ErrorMessage = this.InvalidRequiredMessage;
req.EnableClientScript = this.EnableClientScript;
req.Display = ValidatorDisplay.Dynamic;
req.CssClass = CssClassErr;
Controls.Add(req);
}
CssClass = CssClassTxt;
}
protected override void Render(HtmlTextWriter output)
{
base.Render(output);
if (req != null)
{
req.RenderControl(output);
}
}
}
-
CustomTextBox
public enum TextBoxType
{
Text = 1,
AlphaWithSpace = 2,
AlphaOnly=3,
Numeric=4,
Email = 5,
URL=6
}
public class CustomTextBox : BaseCustomControl
{
public string InvalidDataMessage
{
get
{
string s = "Invalid Data";
object obj = ViewState["InvalidDataMessage"];
if (obj != null)
{
s = (string)obj;
}
return s;
}
set
{
ViewState["InvalidDataMessage"] = value;
}
}
public TextBoxType TextType
{
get
{
TextBoxType t = TextBoxType.AlphaOnly;
object obj = ViewState["TextType"];
if (obj != null)
{
t = (TextBoxType)obj;
}
return t;
}
set
{
ViewState["TextType"] = value;
}
protected override void OnInit(EventArgs e)
{
base.OnInit(e);
reg = new RegularExpressionValidator();
reg.ControlToValidate = this.ID;
reg.ErrorMessage = this.InvalidDataMessage;
reg.EnableClientScript = this.EnableClientScript;
reg.Display = ValidatorDisplay.Dynamic;
//check Type
switch (TextType)
{
case TextBoxType.AlphaOnly:
{
reg.ValidationExpression = @"[a-zA-Z]*";
break;
}
case TextBoxType.Numeric:
{
reg.ValidationExpression = @"[0-9]*";
break;
}
case TextBoxType.AlphaWithSpace:
{
reg.ValidationExpression = @"[a-zA-Z ]*";
break;
}
case TextBoxType.Text:
{
reg.ValidationExpression = @"[\w^\w\W^\W]*";
break;
}
case TextBoxType.Email:
{
reg.ValidationExpression = @"\w+([-+.']\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*";
break;
}
case TextBoxType.URL:
{
reg.ValidationExpression = @"http(s)?://([\w-]+\.)+[\w-]+(/[\w- ./?%&=]*)?";
break;
}
}
Controls.Add(reg);
#endregion
}
protected override void Render(HtmlTextWriter w)
{
base.Render(w);
if (reg != null)
{
reg.RenderControl(w);
}
}
-
DecimalTextBox
public class DecimalTextBox : BaseCustomControl
{
protected override void OnInit(EventArgs e)
{
this.Attributes.Add("onkeypress", "javascript: return AllowDecimal(event,'"+this.ClientID+"');");
base.OnInit(e);
}
protected override void OnPreRender(EventArgs e)
{f(!this.Page.ClientScript.IsClientScriptBlockRegistered("strAllowNumeric"))
{
string script = @"function AllowDecimal(e,txt)
{
var iKeyCode = 0;
if (window.event)
{
iKeyCode = window.event.keyCode
}
else if (e)
{
iKeyCode = e.which;
}
var y = document.getElementById(txt).value;
if(iKeyCode==46 && y.indexOf('.')==-1){ return true;}
else if ((iKeyCode > 47 && iKeyCode < 58)|| iKeyCode==8)
{ return true;}
else
{return false;} };
this.Page.ClientScript.RegisterClientScriptBlock(this.Page.GetType(), "strAllowDecimal", script,true);
}
base.OnPreRender(e);
}
}
}
-
NumricTextBox
public class NumericTextBox : BaseCustomControl
{
protected override void OnInit(EventArgs e)
{ this.Attributes.Add("onkeypress", "javascript: return AllowNumeric(event);");
base.OnInit(e);
protected override void OnPreRender(EventArgs e)
{if(!this.Page.ClientScript.IsClientScriptBlockRegistered("strAllowNumeric"))
{
string script = "function AllowNumeric(e)";
script += "{";
script += "var iKeyCode = 0;";
script += "if (window.event)";
script += "{iKeyCode = window.event.keyCode}";
script += "else if (e)";
script += "{iKeyCode = e.which;}";
script += "if ((iKeyCode > 47 && iKeyCode < 58) || iKeyCode == 8||iKeyCode == 0)";
script += "{ return true}";
script += "else";
script += "{return false;}";
script += "}";
this.Page.ClientScript.RegisterClientScriptBlock(this.Page.GetType(), "strAllowNumeric", script,true);
}
base.OnPreRender(e);
}
catch (Exception ex)
{
throw ex;
}
}
#endregion
}
}
-
StringTextBox
public class StringTextBox : BaseCustomControl
{
public bool AllowAlpha
{
get
{
bool b = true;
object obj = ViewState["AllowAlpha"];
if (obj != null)
{
b = (bool)obj;
}
return b;
}
set
{
ViewState["AllowAlpha"] = value;
}
}
public bool AllowSpace
{
get
{
bool b = false;
object obj = ViewState["AllowSpace"];
if (obj != null)
{
b = (bool)obj;
}
return b;
}
set
{
ViewState["AllowSpace"] = value;
}
}
public bool AllowDigit
{
get
{
bool b = false;
object obj = ViewState["AllowDigit"];
if (obj != null)
{
b = (bool)obj;
}
return b;
}
set
{
ViewState["AllowDigit"] = value;
}
}
protected override void OnInit(EventArgs e)
{
base.OnInit(e);
if ((!AllowAlpha) && AllowDigit)
{
this.Attributes.Add("onkeypress", "javascript: return AllowDigit(event," + AllowSpace.ToString().ToLower() + ");");
}
else if (AllowAlpha)
{
this.Attributes.Add("onkeypress", "javascript: return AllowAlpha(event," + AllowSpace.ToString().ToLower() + "," + AllowDigit.ToString().ToLower() + ");");
}
else
{
this.Enabled = false;
}
protected override void OnPreRender(EventArgs e)
{
string script1="<script language=javascript>";
script1+="function AllowAlpha(e,allowSpace,allowDigit)";
script1+="{";
script1+="var KeyID = (window.event) ? event.keyCode : e.which;";
script1+="if((KeyID >= 65 && KeyID <= 90)";
script1+="|| (KeyID >= 97 && KeyID <= 122)";
script1+="|| (KeyID >= 33 && KeyID <= 47)";
script1+="||(KeyID >= 58 && KeyID <= 64)";
script1+="|| (KeyID >= 91 && KeyID <= 96)";
script1+="|| (KeyID ==32 && allowSpace)";
script1+="|| (KeyID > 47 && KeyID < 58 && allowDigit)";
script1+="|| (KeyID >= 123 && KeyID <= 126))";
script1+="{return true;}";
script1+="else";
script1+="{return false;}";
script1+="}";
script1+="</script>";
this.Page.ClientScript.RegisterClientScriptBlock(this.Page.GetType(), "strAllowAlpha", script1);
if ((!AllowAlpha) && AllowDigit)
{
string script="<script language=javascript>";
script+="function AllowDigit(e,allowSpace)";
script+="{";
script+="var iKeyCode = 0;";
script+="if (window.event)";
script+="{iKeyCode = window.event.keyCode}";
script+="else if (e)";
script+="{iKeyCode = e.which;}";
script+="if ((iKeyCode > 47 && iKeyCode < 58)";
script+="|| (iKeyCode ==32 && allowSpace))";
script+="{ return true}";
script+="else";
script+="{return false;}";
script+="}";
script += "</script>";
this.Page.ClientScript.RegisterClientScriptBlock(this.Page.GetType(), "strAllowDigit", script);
}
base.OnPreRender(e);
}
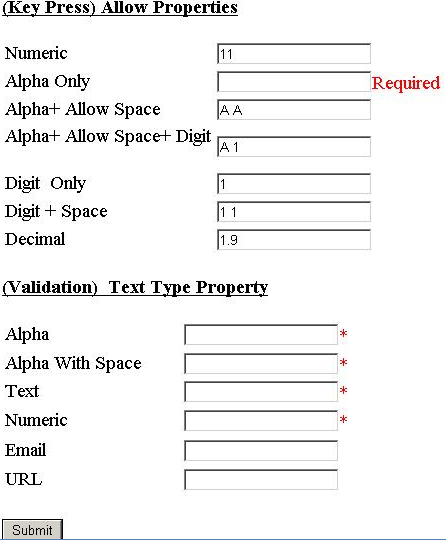