Before explaining the primary subject of this article we will introduce the function which will be used in our article.
Clipboard.ContainsImage():
- First of all it returns a Boolean type that means either "true" or "false".
- Indicates whether there is data on the clipboard that is in the System.Windows.Forms.DataFormats.Bitmap format or that can be converted to that format.
Clipboard.GetImage():
- It returns the image itself.
- Retrieves the image from the clipboard.
PictureBoxSizeMode:
- It is used to specify how an image is positioned within a System.Windows.Forms.PictureBox.
- There are a total of five possible types of "SizeMode" for the "Picturebox" Control.
o Normal
o StretchImage
o AutoSize
o CenterImage
o Zoom
- For more understanding of the "SizeModes" see the images below:
pictureBox1.SizeMode = PictureBoxSizeMode.Normal;
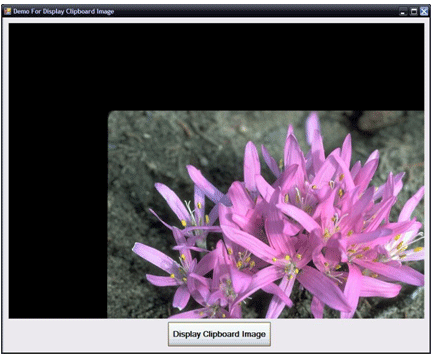
pictureBox1.SizeMode = PictureBoxSizeMode.StretchImage;
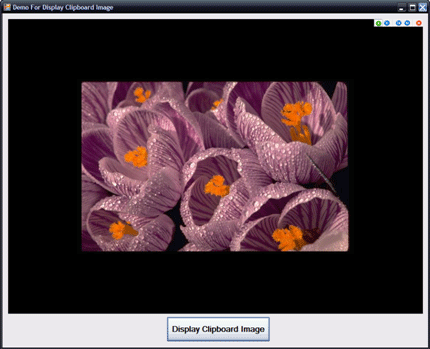
pictureBox1.SizeMode = PictureBoxSizeMode.AutoSize;
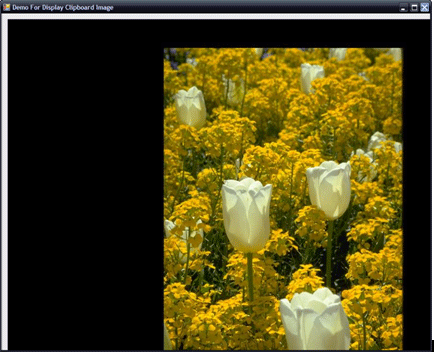
pictureBox1.SizeMode = PictureBoxSizeMode.CenterImage;
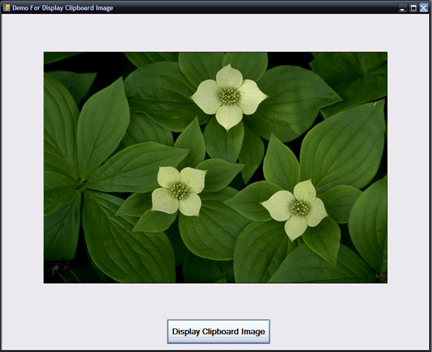
pictureBox1.SizeMode = PictureBoxSizeMode.Zoom;
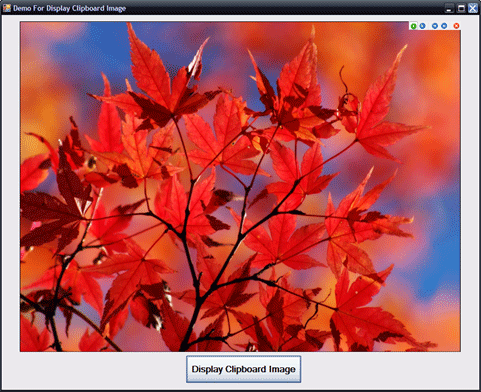
Code :
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace DisplayClipboardImage
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
if (Clipboard.ContainsImage())
{
pictureBox1.SizeMode = PictureBoxSizeMode.StretchImage;
pictureBox1.Image = Clipboard.GetImage();
}
else
{
MessageBox.Show("Clipboard is empty. Please Copy Image.");
}
}
}
}