In this article, we will see how we can add a search bar to the TableView control. TableView is a very important and most widely used control. Thus, we will be having a lot of the tutorials that focus on the different aspects of it. This is the third one of the TableView series. The others are:
Ingredients
- Visual Studio 2012 or higher / Xamarin Studio 5.
- Xamarin iOS 6.4.5 or higher.
- Mac OS X Yosemite(10.10) & above.
Procedure
- Create a new Application in Xamarin Studio by selecting New Solution from the welcome screen.
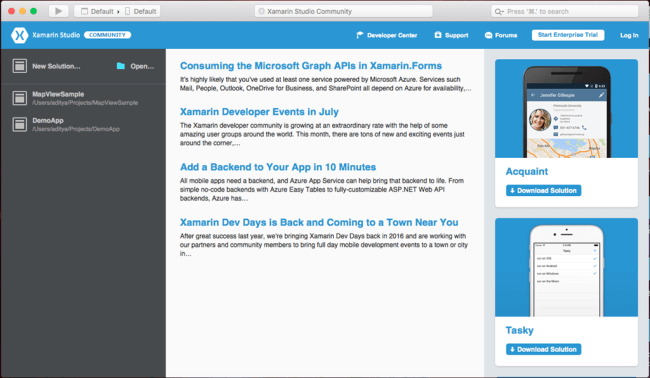
- Select iOS app from the installed templates. Now, as you can see, we have a lot of options, like Single View app, Master-Detail app, Tabbed app. These are nothing but pre-defined UI screens for the ease-of-use of the developers. For this tutorial, we will select Single View app.
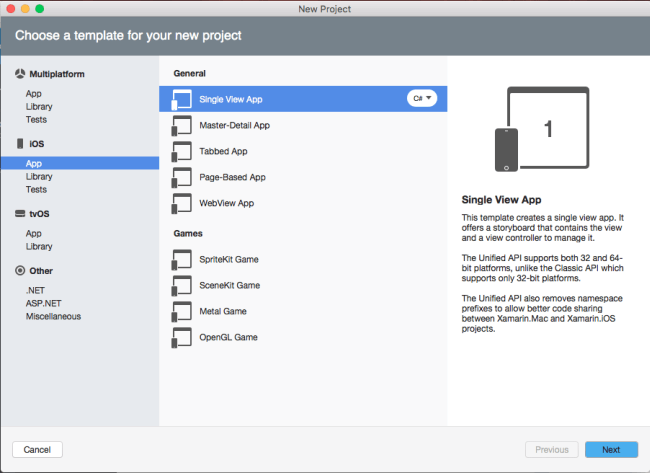
- Now, we will name the app and select the minimum iOS version that our app will support, as shown here.
![version]()
- Now, we will name the project, give the location for the solution to be saved, and select the version control for git (if required), as shown below:
![location]()
- Now, we can see that the solution file is created and it contains all the required files, as shown below.
![solution]()
- Now, we will open ViewController.cs from the solution and import CoreGraphics, System, System.Collections.Generic, and UIKit.
- using System;
- using CoreGraphics;
- using System.Collections.Generic;
- using UIKit;
- Create an object of UITableView, TableSource, UISearchBar and List<TableItem> in the ViewController class.
- UITableView table;
- TableSource tableSource;
- List<TableItem> tableItems;
- UISearchBar searchBar;
- To set the title of the app in the ViewDidLoad function, just write the following:
- Title = "SearchBarWithTableView Sample";
- Now, we will add the TableView properties in the ViewDidLoad function. Also, add the TableView to the View and the SearchBar to the TableHeaderView. Finally, our ViewDidLoad() will look, as given below:
- protected ViewController(IntPtr handle) : base(handle)
- {
-
- }
-
- public override void ViewDidLoad()
- {
- base.ViewDidLoad();
-
-
-
- searchBar = new UISearchBar();
- searchBar.SizeToFit();
- searchBar.AutocorrectionType = UITextAutocorrectionType.No;
- searchBar.AutocapitalizationType = UITextAutocapitalizationType.None;
- searchBar.TextChanged += (sender, e) =>
- {
-
- searchTable();
- };
-
- Title = "SearchBarWithTableView Sample";
- table = new UITableView(new CGRect(0, 20, View.Bounds.Width, View.Bounds.Height - 20));
-
- tableItems = new List<TableItem>();
-
- tableItems.Add(new TableItem("Vegetables") { ImageName = "Vegetables.jpg" });
- tableItems.Add(new TableItem("Fruits") { ImageName = "Fruits.jpg" });
- tableItems.Add(new TableItem("Flower Buds") { ImageName = "Flower Buds.jpg" });
- tableItems.Add(new TableItem("Legumes") { ImageName = "Legumes.jpg" });
- tableItems.Add(new TableItem("Tubers") { ImageName = "Tubers.jpg" });
- tableSource = new TableSource(tableItems);
- table.Source = tableSource;
- table.TableHeaderView = searchBar;
- Add(table);
- }
- We also add the searchTable() method for the UISearchBar to perform the search action.
- private void searchTable()
- {
-
- tableSource.PerformSearch(searchBar.Text);
- table.ReloadData();
- }
- Now, we will add a new class, TableSource, to the solution.
![class]()
- Once the file is added, open TableSource.cs and add the code, as given below:
- using System;
- using System.Collections.Generic;
- using System.IO;
- using System.Linq;
- using Foundation;
- using UIKit;
-
- namespace SearchBarWithTableView
- {
- public class TableSource : UITableViewSource
- {
- private List<TableItem> tableItems = new List<TableItem>();
- private List<TableItem> searchItems = new List<TableItem>();
- protected string cellIdentifier = "TableCell";
-
- public TableSource(List<TableItem> items)
- {
- this.tableItems = items;
- this.searchItems = items;
- }
-
- public override nint RowsInSection(UITableView tableview, nint section)
- {
- return searchItems.Count;
- }
-
- public override UITableViewCell GetCell(UITableView tableView, NSIndexPath indexPath)
- {
-
- UITableViewCell cell = tableView.DequeueReusableCell(cellIdentifier);
-
-
- var cellStyle = UITableViewCellStyle.Default;
-
-
- if (cell == null)
- {
- cell = new UITableViewCell(cellStyle, cellIdentifier);
- }
-
- cell.TextLabel.Text = searchItems[indexPath.Row].Title;
- cell.ImageView.Image = UIImage.FromFile("Images/" + searchItems[indexPath.Row].ImageName);
-
- return cell;
- }
-
- public override nint NumberOfSections(UITableView tableView)
- {
- return 1;
- }
-
- public void PerformSearch(string searchText)
- {
- searchText = searchText.ToLower();
- this.searchItems = tableItems.Where(x => x.Title.ToLower().Contains(searchText)).ToList();
- }
- }
- }
This class is used to implement the abstract class UITableViewSource. Thus, we define the basic functions required for a TableView. RowsInSection is used to determine the number of rows present in a section; NumberOfSections are used to determine the number of sections; the TableView is divided and the GetCell defines the UITableViewCell that will be rendered for a particular row. We also add methods which help in depicting the Search in action by adding a PerformSearch() method.
- Now, we will add another class, TableItem, to the solution, for the customized cell style. Once the file is added, open TableItem.cs and add the code, as given below:
- using System;
- using UIKit;
-
- namespace SearchBarWithTableView
- {
- public class TableItem
- {
- public string Title { get; set; }
-
- public string ImageName { get; set; }
-
- public UITableViewCellStyle CellStyle
- {
- get { return cellStyle; }
- set { cellStyle = value; }
- }
- protected UITableViewCellStyle cellStyle = UITableViewCellStyle.Default;
-
- public UITableViewCellAccessory CellAccessory
- {
- get { return cellAccessory; }
- set { cellAccessory = value; }
- }
- protected UITableViewCellAccessory cellAccessory = UITableViewCellAccessory.None;
-
- public TableItem() { }
-
- public TableItem(string title)
- { Title = title; }
-
- }
- }
- Now, we run the app on the iPhone simulator of our choice (I did it on iPhone 4S) and it looks, as shown below.
![simulator]()
- Start typing the word that you want to search.
Make some beautiful apps using Xamarin.iOS and tweet your queries to @adiiaditya. If you want to fork this project, visit my Git.