Unit testing is a crucial aspect of software development that involves testing individual units of code, typically functions or methods, to ensure they behave as expected. In C#, you can write unit tests using various testing frameworks, including:
- NUnit: A popular testing framework for .NET that provides a rich set of features and a user-friendly API.
- xUnit: Another widely used testing framework that offers a more modern and flexible approach to unit testing.
When unit testing in C#, you can use libraries like Moq or NSubstitute to create mock objects and isolate dependencies, making your tests more efficient and reliable. NUnit is a popular, open-source unit testing framework for C# and other .NET languages, initially ported from JUnit, used for writing and executing automated tests to ensure code quality.
Here, we are implementing basic CRUD operation in web API using C#, and then we are enlisting the different test cases for all those operations and writing a code to test those scenarios using the NUnit framework.
Pre-requisite
1. Open VS 2022
2. Create a Web API project
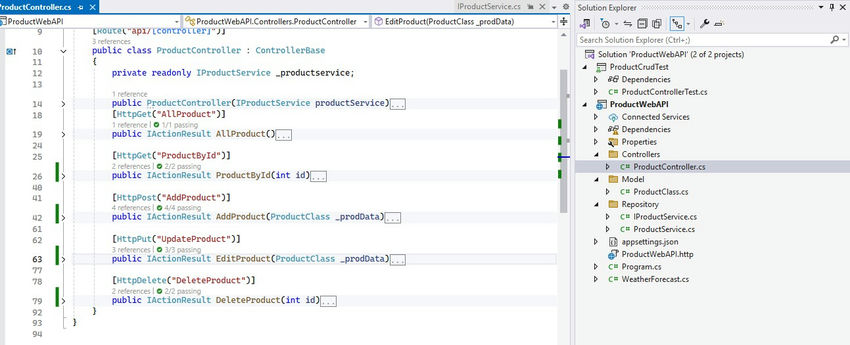
3. Add NUnit Test Project
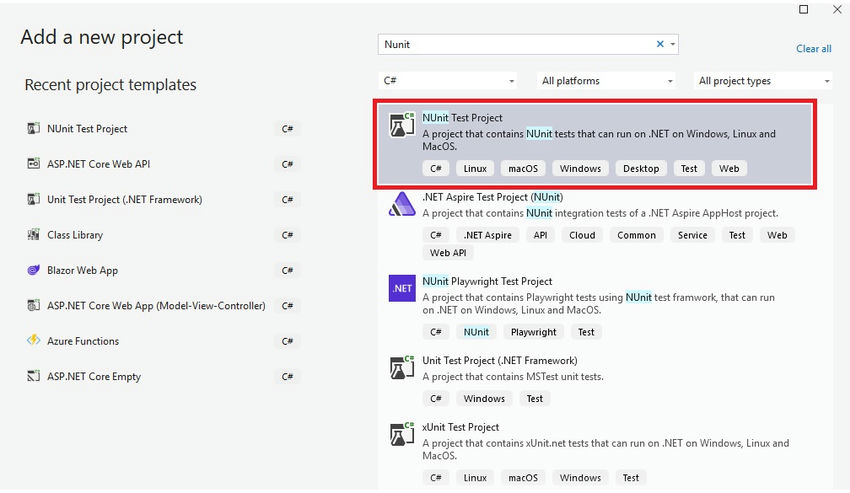
4. Install required packages from NuGet Package
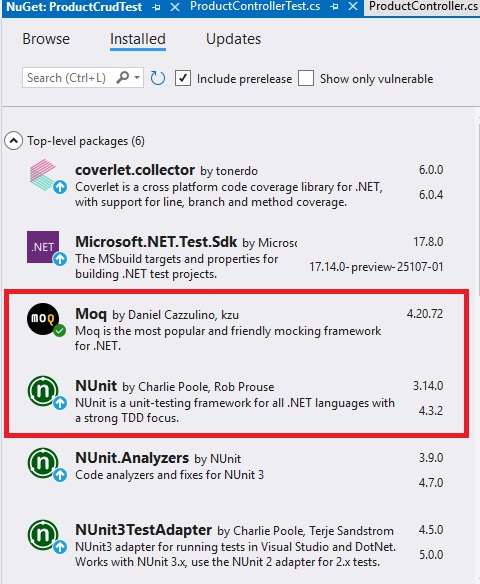
Core Features of NUnit
NUnit framework has extensive tools for streamlining unit testing, including accurate code verification, logical test design, and broad test coverage. Let's look at three crucial aspects for building good tests.
NUnit Test Lifestyle Attributes
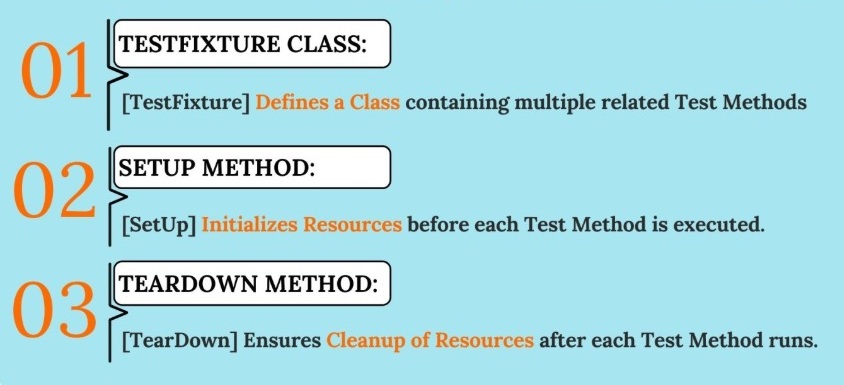
- [Test Fixture]: is a class or function that has the [TestFixture] attribute, which indicates that it contains tests.
- [SetUp]: This method initializes resources.
- [Test]: It contains the test logic.
- [TearDown]: It assures cleanup after each test.
Now, list down all possible test case scenarios w.r.t the web API and create a test method for each of them.
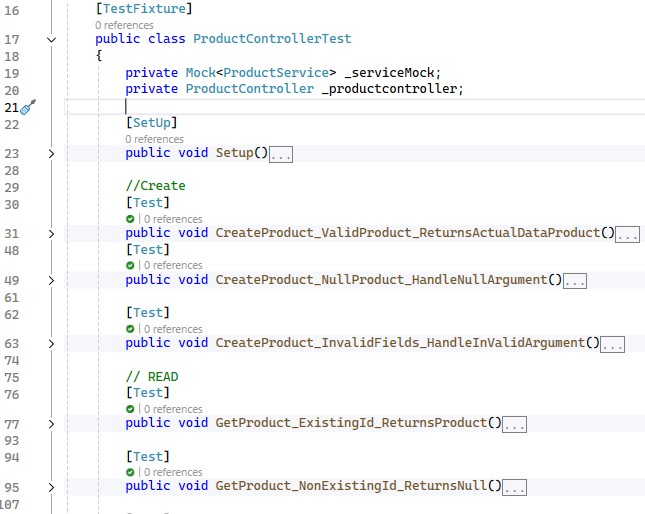
In each test method, we need to follow the 3A pattern of the NUnit Framework i.e. AAA[Arrange-Act-Assert]. It is a common way to structure unit tests to make them more readable and organized.
- Arrange: Set up everything needed for the test—objects, variables, mock data, etc.
- Act: Perform the actual action that you want to test.
- Assert: Verify that the outcome is what you expected.
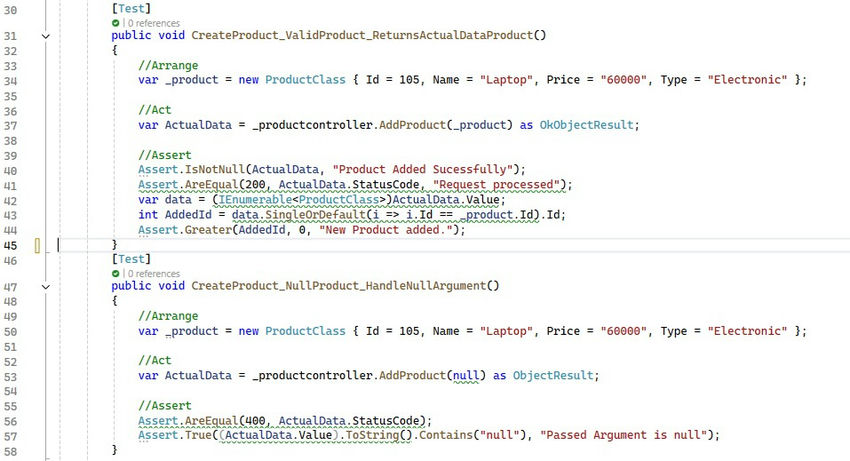
Once we have done coding on all possible scenarios, then we can run the NUnit project by executing the “Run All Test” option.
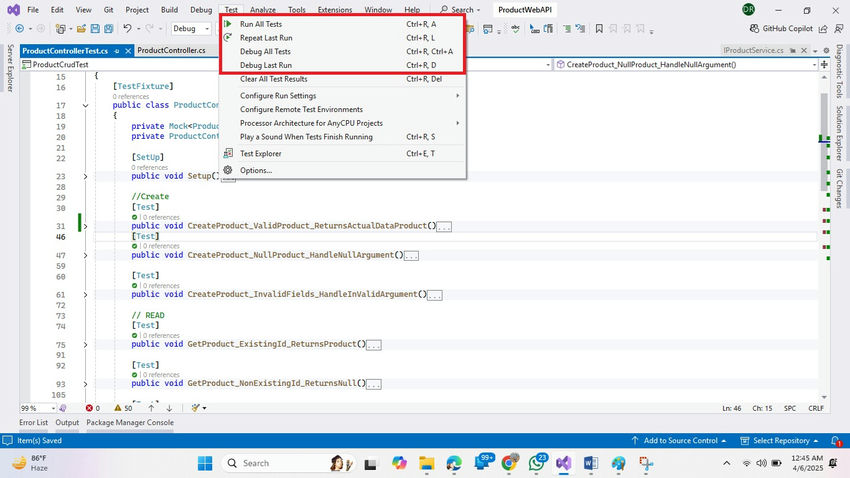
We can run all test cases at a single time, and for complex test cases, we can also debug at a breakpoint by opting for “Debug All Tests”.
On running all test cases, a Test Explorer will open a listing of all test methods as a monitoring window, which depicts the result of all test cases.
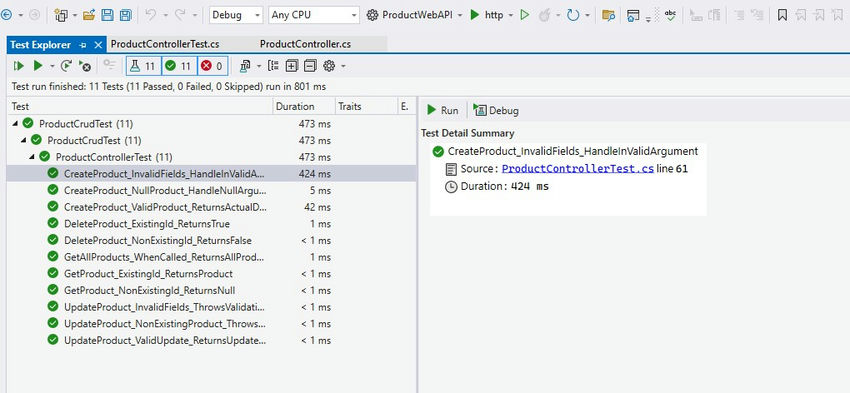
If any scenario doesn’t meet the required criteria or expected results, then it will be indicated in the test explorer in red color with the reasonable issue and its possible solution.
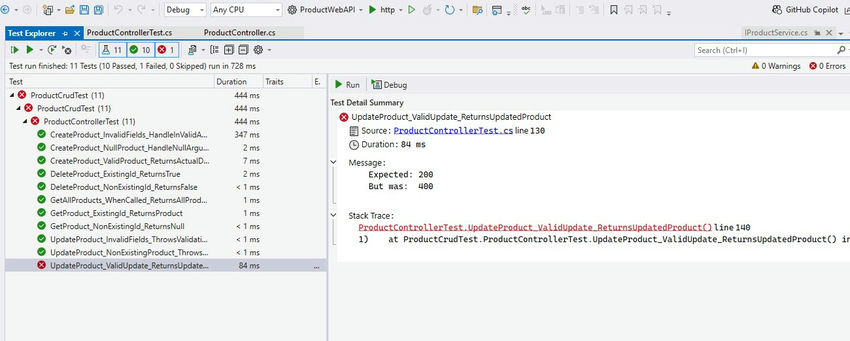
In this article, we've explored the key features of NUnit testing, from understanding the basics of unit testing to setting up and using the NUnit framework for your.NET unit testing applications. You may efficiently validate your code by learning how to build simple unit tests, leverage test assertions, and arrange them with test fixtures.
NUnit's adaptability makes it a powerful tool for unit testing in software engineering, whether concentrating on isolated components or complicated situations. Using NUnit testing tools and rigorous testing techniques can lead to high-quality, bug-free code in your apps.