CSRF is a type of web security vulnerability that forces authenticated users to submit unwanted requests to a web application.
It's a way for attackers to manipulate users into performing actions they didn't intend to, often leading to data theft, unauthorized actions, or even complete account compromise.
Simple ASP.NET Core Web Application
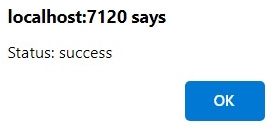
It has two buttons.
- "Card Details": Uses form to access card details.
- "Make Ajax Call": Access card details with Ajax call.
Here is a simple controller to access user card details.
- The [ValidateAntiForgeryToken] attribute ensures that any request to this action method must include a valid anti-forgery token.
- Enable Antiforgery in ASP.NET Core: Antiforgery middleware is added to the Dependency injection container when one of the following APIs is called in the Program. cs.
- AddMvc
- MapRazorPages
- MapControllerRoute
- AddRazorComponents
- Customize Antiforgery Token: We can customize the anti-forgery token by configuring the anti-forgery options in the Program.cs file.
- Implement Antiforgery token with Cookie: This should now protect your endpoints that are using POST, PUT, and DELETE HTTP verbs.
- Make AJAX request: This approach helps in situations where forms aren't directly used, like when working with APIs or SPAs (Single Page Applications).
Output
![Output]()
Summary
- CSRF attacks trick authenticated users into making requests that they did not intend.
- ASP.NET Core protects against CSRF by using anti-forgery tokens, which must be validated on the server for every POST request.
- For AJAX requests, the token needs to be included in the request headers.
You can access the sample project here GitHub