VB .NET does not have an AutoRedraw property for forms
This is a problem if you wish to display text and graphics directly on a form. This brief project should help to provide you with AutoRedraw capability.
The theory is as follows
- Create a bitmap from your form at startup.
- Write your graphics and text to the bitmap (not the form).
- Copy the bitmap to the form to show the graphics.
- Copy the bitmap to the form to repaint.
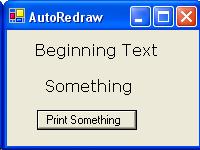
Some explanation
If you try to print directly to the form first and then save each printing from the form to the bitmap you will run into problems since the system may not display your graphics in time to be copied. You could try the 'System.Windows.Forms.Application.DoEvents()' statement, but an intervening Paint event might spoil everything.
Here is the code
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
- Private b1 As Bitmap
-
- Private g1 As Graphics
- Private Sub Form1_Activated(ByVal sender As Object, _ByVal e As System.EventArgs) Handles MyBase.Activated
- Static done As Boolean = False
- If Not done Then
-
- Size = New Size(200, 150)
- Text = "AutoRedraw"
-
- With Button1
- .Text = "Print Something"
- .Size = New Size(100, 20)
- .Location = New Point(30, 80)
- End With
-
-
- b1 = New Bitmap(Width, Height, Me.CreateGraphics())
-
-
-
-
- g1 = Graphics.FromImage(b1)
-
- done = True
-
-
- FormPrint("Beginning Text", 25, 10)
- End If
- End Sub
-
- Private Sub Button1_Click(ByVal sender As System.Object, _ByVal e As System.EventArgs) Handles Button1.Click
- FormPrint("Something", 35, 46)
- End Sub
- Private Sub FormPrint(ByVal t$, ByVal x1%, ByVal y1%)
-
-
- g1.DrawString(t, New Font("Verdana", 12), _Brushes.Black, x1, y1)
-
- Me.CreateGraphics.DrawImage(b1, 0, 0)
- End Sub
- Private Sub Form1_Paint( _ByVal sender As Object, _ByVal e As System.Windows.Forms.PaintEventArgs) _Handles MyBase.Paint
-
- e.Graphics.DrawImage(b1, 0, 0)
- End Sub