Hi, I am trying to deserilize the below sample json, however, it seems that the list doesn't hold all the data in one object. Please advise.
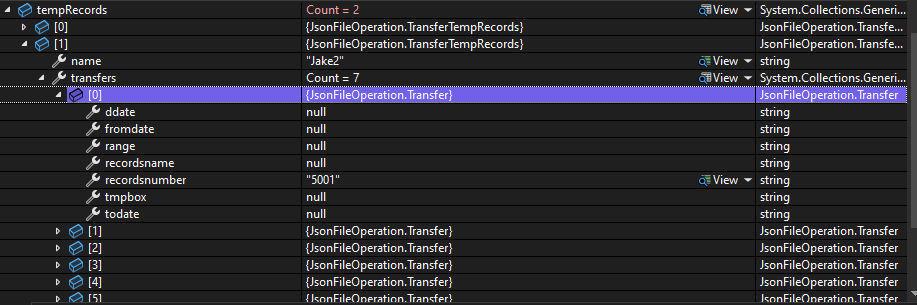
internal class Program
{
static void Main(string[] args)
{
string jsonString = @"[
{
""userid"": ""0001"",
""name"": ""Jake1"",
""transfers"": [
{ ""recordsnumber"": ""5001"" },
{ ""recordsname"": ""test"" },
{ ""tmpbox"": ""5005"" },
{ ""ddate"": ""10/23/2024"" },
{ ""fromdate"": ""10/23/2024"" },
{ ""todate"": ""10/23/2024"" },
{ ""range"": ""5004"" }
]
},
{
""userid"": ""0002"",
""name"": ""Jake2"",
""transfers"": [
{ ""recordsnumber"": ""5001"" },
{ ""recordsname"": ""test"" },
{ ""tmpbox"": ""5005"" },
{ ""ddate"": ""10/23/2024"" },
{ ""fromdate"": ""10/23/2024"" },
{ ""todate"": ""10/23/2024"" },
{ ""range"": ""range"" }
]
}
]";
List<TransferTempRecords> tempRecords = ParseJsonFromString(jsonString);
}
public static List<TransferTempRecords> ParseJsonFromString(string jsonString)
{
try
{
return JsonSerializer.Deserialize<List<TransferTempRecords>>(jsonString);
}
catch (JsonException ex)
{
Console.WriteLine($"Error parsing JSON: {ex.Message}");
return null;
}
}
}
public class TransferTempRecords
{
public string userid { get; set; }
public string name { get; set; }
public List<Transfer> transfers { get; set; }
}
public class Transfer
{
public string recordsnumber { get; set; }
public string recordsname { get; set; }
public string tmpbox { get; set; }
public string ddate { get; set; }
public string fromdate { get; set; }
public string todate { get; set; }
public string range { get; set; }
}