<%@ Import Namespace="System.Web.Mail" %>
<script language="c#" runat="server">
private void btnSend_Click(object sender, System.EventArgs e)
{
MailMessage msg = new MailMessage();
msg.To = txtTo.Text;
msg.From = txtFrom.Text;
msg.Subject = txtSubject.Text;
msg.Body = txtContent.Value;
lblStatus.Text = "Sending...";
SmtpMail.Send(msg);
lblStatus.Text = "Sent email (" + txtSubject.Text + ") to " +txtTo.Text;
}
</script>
<html>
<body>
<h3>
Email From ASP.NET</h3>
<form id="MailForm" method="post" runat="server">
<asp:Label ID="Label1" Style="left: 100px; position: absolute; top: 100px" runat="server">From:
</asp:Label>
<asp:TextBox ID="txtFrom" Style="left: 200px; position: absolute; top: 100px"
runat="server"></asp:TextBox>
<asp:RequiredFieldValidator ID="FromValidator1" Style="left: 100px; position: absolute;
top: 375px" runat="server" ErrorMessage="Please Enter the Email From." Width="200px"
Height="23px" ControlToValidate="txtFrom"></asp:RequiredFieldValidator>
<asp:RegularExpressionValidator ID="FromValidator2" Style="left: 100px; position: absolute;
top: 400px" runat="server" ErrorMessage="Please Enter a Valid From Email address"
ControlToValidate="txtFrom" ValidationExpression="\w+([-+.]\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)
*"></asp:RegularExpressionValidator>
<asp:Label ID="Label2" Style="left: 100px; position: absolute; top: 125px" runat="server">To:
</asp:Label>
<asp:TextBox ID="txtTo" Style="left: 200px; position: absolute; top: 125px"
runat="server"></asp:TextBox>
<asp:RequiredFieldValidator ID="ToValidator1" Style="left: 100px; position: absolute;
top: 425px" runat="server" ErrorMessage="Please Enter the Email To." Width="200px"
Height="23px" ControlToValidate="txtTo"></asp:RequiredFieldValidator>
<asp:RegularExpressionValidator ID="ToValidator2" Style="left: 100px; position: absolute;
top: 450px" runat="server" ErrorMessage="Please Enter a Valid To Email address"
ControlToValidate="txtTo" ValidationExpression="\w+([-+.]\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)
*"></asp:RegularExpressionValidator>
<asp:Label ID="Label3" Style="left: 100px; position: absolute; top: 150px"
runat="server">Subject</asp:Label>
<asp:TextBox ID="txtSubject" Style="left: 200px; position: absolute; top: 150px"
runat="server"></asp:TextBox>
<asp:Label ID="Label4" Style="left: 100px; position: absolute; top: 175px" runat="server">Mail:
</asp:Label>
<textarea runat="server" id="txtContent" style="left: 200px; width: 400px; position: absolute;
top: 175px; height: 125px" rows="7" cols="24">
</textarea>
<asp:Button ID="btnSend" Style="left: 200px; position: absolute; top: 350px" runat="server"
Text="Send" OnClick="btnSend_Click"></asp:Button>
<asp:Label ID="lblStatus" Style="left: 250px; position: absolute; top: 350px" runat="server">
</asp:Label>
</form>
</body>
</html>
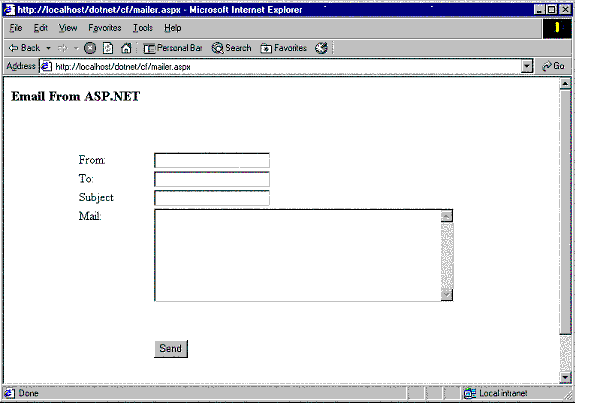
Figure 1 : Email Form in action
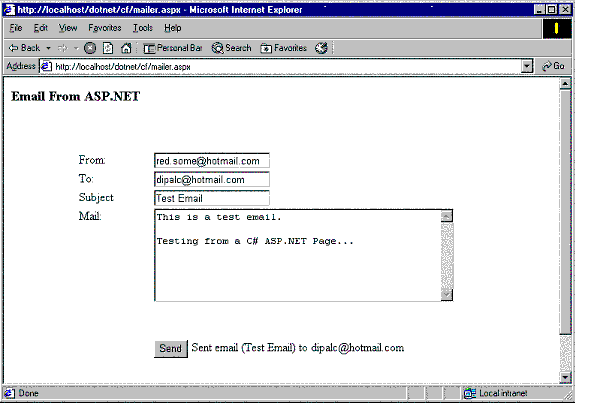
Figure 2: Results
In order to specify another SMTP server , use the SmtpServer field of the SmtpMail object as shown in figure 2 :
Listing 2: Use another mail server
private void btnSend_Click(object sender, System.EventArgs e)
{
MailMessage msg = new MailMessage();
msg.To = txtTo.Text;
msg.From = txtFrom.Text;
msg.Subject = txtSubject.Text;
msg.Body = txtContent.Value;
lblStatus.Text = "Sending...";
SmtpMail.SmtpServer = "smtp.yourISP.com";
SmtpMail.Send(msg);
lblStatus.Text = "Sent email (" + txtSubject.Text + ") to " + txtTo.Text;
}
Here is a code snippet for adding attachments :
Listing 3: Send Attachments
{
....
StrFilePath = "c:\\emps.txt";
MailAttachment attach = new MailAttachment(strFilePath);
msg.Attachments.Add(attach);
....
}
Go ahead - Email enable your web pages !