Description:
This article will show you operations on TreeView using Database. It will show you mainly three kinds of operations such as Add, Update & Delete; then after performing those operations the effect will immediately be shown in the Treeview & the GridView.
I have written two main functions; the first one is for adding data into a TreeView from the database & the second function is for adding data into DataGridView from the database.
private void BindDataInTreeView()
{
MyTreeView.Nodes.Clear();
try
{
conn.Open();
//It will show Name of the Students into TreeView.
SqlCommand cmd = new SqlCommand("SELECT StudentName FROM Student", conn);
dr = cmd.ExecuteReader();
while (dr.Read())
{
//Here "Node" Means It Will Add Nodes As All Root Nodes...
MyTreeView.Nodes.Add(dr.GetValue(0).ToString());
}
dr.Close();
}
catch (Exception ex)
{
MessageBox.Show(ex.ToString());
}
finally
{
conn.Close();
}
}
private void BindDataInGridView()
{
try
{
conn.Open();
//It will show all data of Students into DataGridView.
SqlCommand cmd = new SqlCommand("SELECT * FROM Student", conn);
DataSet ds = new DataSet();
SqlDataAdapter da = new SqlDataAdapter(cmd);
da.Fill(ds);
MyDataGridView.DataSource = ds.Tables[0];
dr.Close();
}
catch (Exception ex)
{
MessageBox.Show(ex.ToString());
}
finally
{
conn.Close();
}
}
"Student" Table Data Definition :
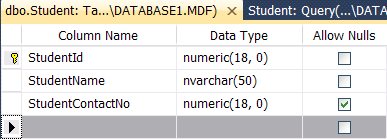
Data which I have stored:
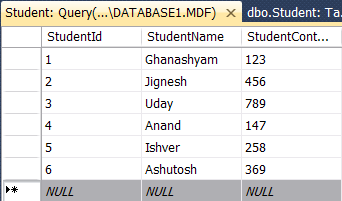
When you will run this it will show you a window like below:
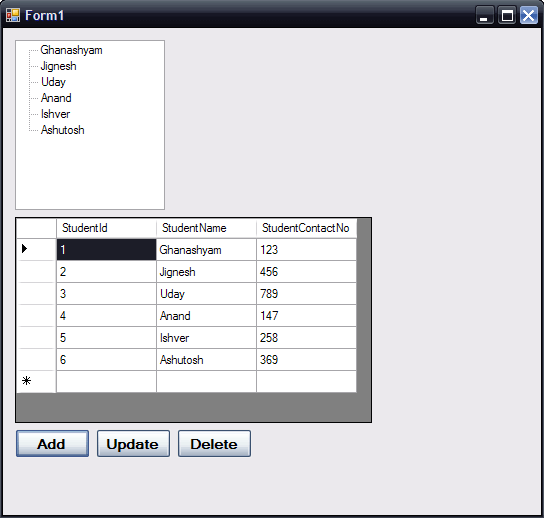
In the above window it will display a student's name in the TreeView & all data into the DataGridView in the form load event.
private void Form1_Load(object sender, EventArgs e)
{
BindDataInTreeView();
BindDataInGridView();
}
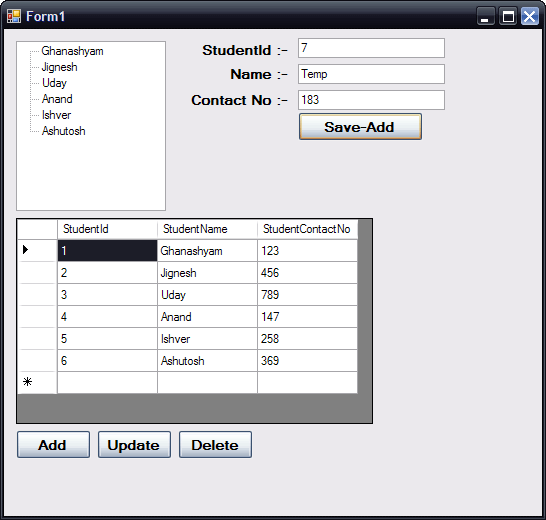
private void btnSaveAdd_Click(object sender, EventArgs e)
{
if ((txtStudId.Text != null) && (txtStudName.Text != null))
{
try
{
conn.Open();
SqlCommand cmd = new SqlCommand("Insert Into Student Values(" + txtStudId.Text + ",'" + txtStudName.Text + "'," + txtStudContactNo.Text + ");", conn);
cmd.ExecuteNonQuery();
MessageBox.Show("Successfully Insert Your Record In Database.");
NotVisibleAll();
btnSaveAdd.Visible = false;
conn.Close();
BindDataInTreeView();
BindDataInGridView();
}
catch (Exception ex)
{
MessageBox.Show(ex.ToString());
}
finally
{
conn.Close();
}
}
else
{
MessageBox.Show("Please Fill Up Values In Blank Field.");
}
}
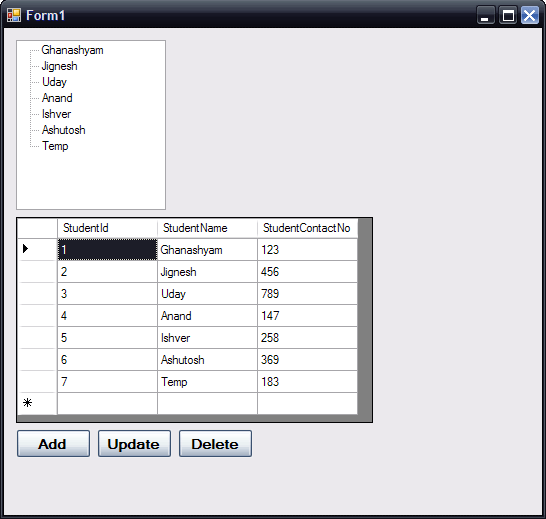
When you click the "Add" button then it will show you textboxes & then you can add new data into the database. Once you have added new data then it will automatically add that new entry in both TreeView & DataGridView.
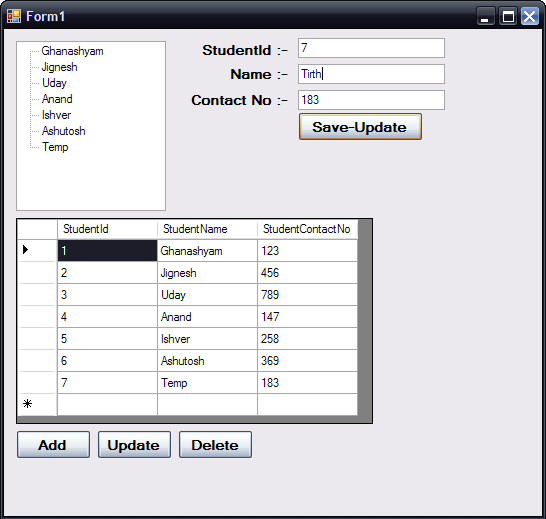
private void btnSaveUpdate_Click(object sender, EventArgs e)
{
if ((txtStudId.Text != null) && (txtStudName.Text != null))
{
try
{
conn.Open();
SqlCommand cmd = new SqlCommand("Update Student Set StudentName='" + txtStudName.Text + "',
StudentContactNo=" + txtStudContactNo.Text + " WHERE StudentId=" + txtStudId.Text + ";", conn);
cmd.ExecuteNonQuery();
MessageBox.Show("Successfully Updated Your Record In Database.");
NotVisibleAll();
btnSaveUpdate.Visible = false;
conn.Close();
BindDataInTreeView();
BindDataInGridView();
}
catch (Exception ex)
{
MessageBox.Show(ex.ToString());
}
finally
{
conn.Close();
}
}
else
{
MessageBox.Show("Please Fill Up Values In Blank Field.");
}
}
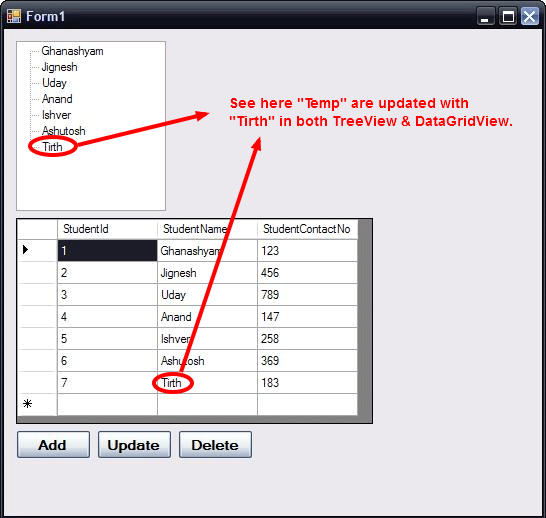
When you click the "Update" button it will show you textboxes & then you can update data into the database. Once you have updated data then it will automatically be updated in both TreeView & DataGridView.
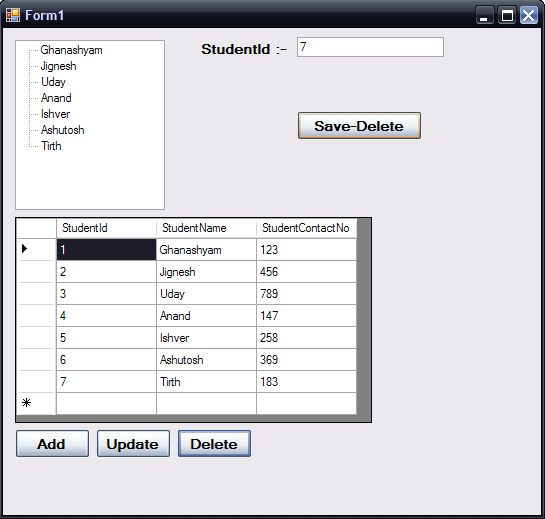
private void btnSaveDelete_Click(object sender, EventArgs e)
{
if (txtStudId.Text != null)
{
try
{
conn.Open();
SqlCommand cmd = new SqlCommand("DELETE FROM Student WHERE StudentId=" + txtStudId.Text + ";", conn);
cmd.ExecuteNonQuery();
MessageBox.Show("Successfully Deleted Your Record In Database.");
lblStudId.Visible = false;
txtStudId.Visible = false;
btnSaveDelete.Visible = false;
conn.Close();
BindDataInTreeView();
BindDataInGridView();
}
catch (Exception ex)
{
MessageBox.Show(ex.ToString());
}
finally
{
conn.Close();
}
}
else
{
MessageBox.Show("Please Fill StudentId Field.");
}
}
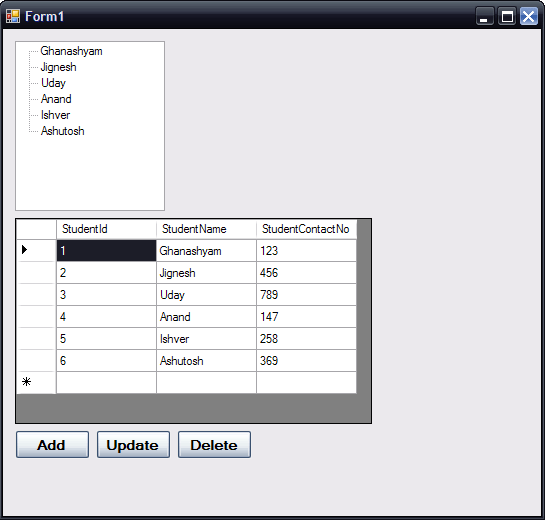
When you click on "Delete" button then it will show you textboxes & then you can Delete data from database. Once you deleted Data then it will automatically delete in both TreeView & DataGridView.